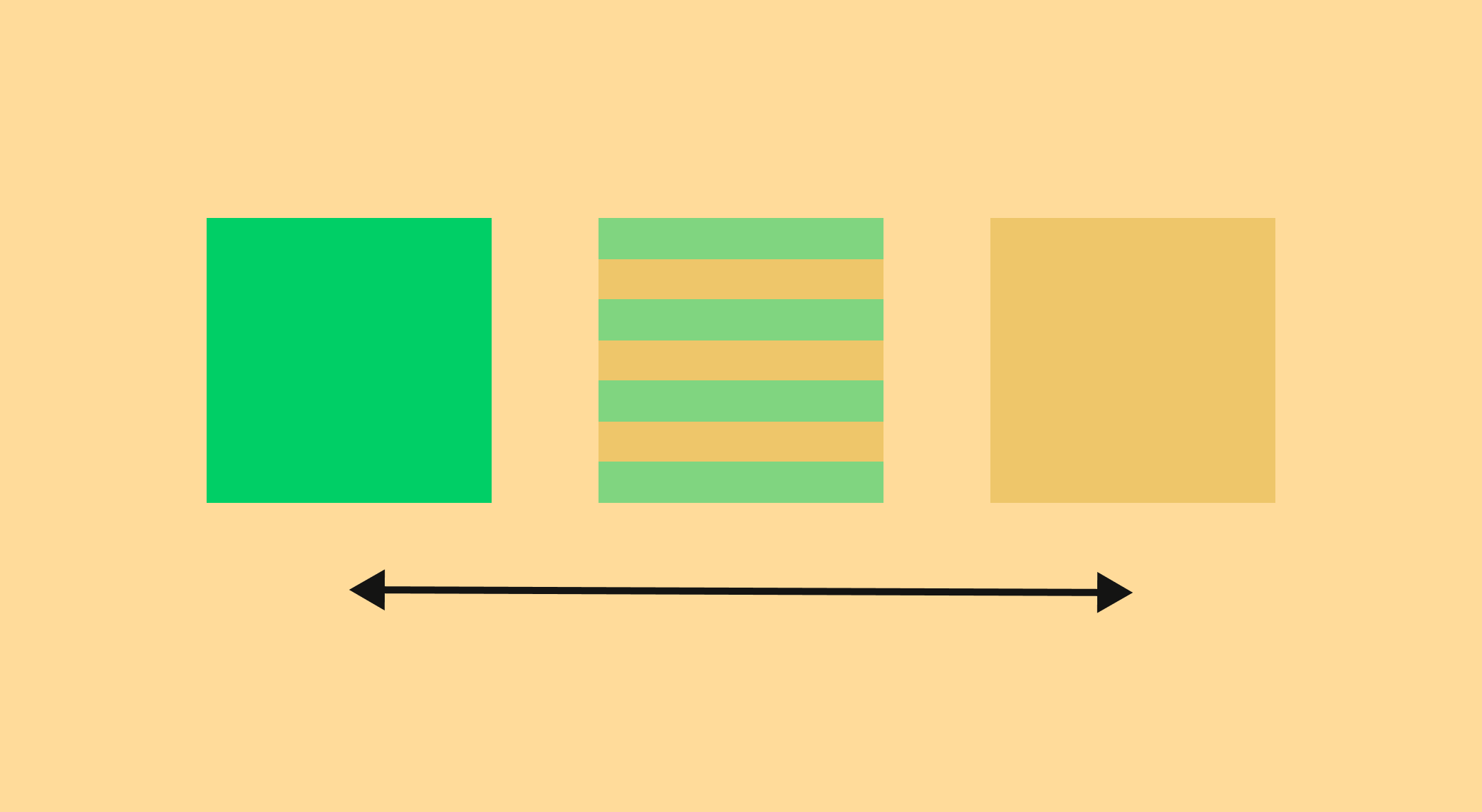
View Transition API
A short recap
13 Apr 2025
The new View Transition API is now supported in Chrome, Edge, Opera and Safari, so I suppose it’s about time I learned how to use it.
As with any new API, there is a million ways to do things. This post is me trying to reduce it to a minimum.
What can it do?
It took me a while to figure this out, mostly because the examples i found could have been achieved with css transitions (like toggling light/dark mode).
The main thing this API can do that was impossible before is basically:Animate between two different DOM states.
Or, more explicitly:
The View Transition API lets you animate between two different DOM states by smoothly transitioning elements that change, move, appear, or disappear.
Basic example
You can literally animate between two classes.
<div class="container">
<ul id="view" class="view grid">
<li>A</li>
<li>B</li>
<li>C</li>
</ul>
<button id="button">Toggle</button>
</div>
<script>
const button = document.getElementById('button');
const view = document.getElementById('view');
// Add or remove "grid" class on list
button.onclick = () => {
document.startViewTransition(() => {
view.classList.toggle('grid');
});
};
</script>
Here’s what that looks like. The default transition is a fade:
“That’s it?” you say.
Well, this just fades from one DOM state to another. We could tell the browser to animate individual elements, like the list items..
Let’s add a view transition name to each list item (these have to be unique):
<ul id="view" class="view grid">
<li style="view-transition-name: item-a;">A</li>
<li style="view-transition-name: item-b;">B</li>
<li style="view-transition-name: item-c;">C</li>
</ul>
Which gives us this:
These identifiers are used to match the elements between the two states, or even two pages..
Page to page transition
First of all, to add view transitions to page changes you don’t really need any javascript..
@view-transition { navigation: auto;}
This will automatically fade pages in and out on navigation.
In addition, you can add view transition names to elements to animate them from one page to another.
This is what I’ve done for the blog posts on this site, where the post thumbnail is transitioned when navigating to the post.
Old page:
<img src="/thumbnail.png" style="view-transition-name:view-transition-api-image" />
New page:
<img src="/full.png" style="view-transition-name:view-transition-api-image" />
Result:
What should i use this for?
Well, I don’t know what’s right for you, but I for one shall:
- Use
startViewTransition
instead ofreact-transition-group
. - Add gentle view transitions to future projects using
@view-transition
. - Weigh up using this whenever i start absolutely positioning elements to animate them.
Summary of what’s new
TL;DR:
document.startViewTransition(callback)
- A new JavaScript method.
- Wrap your DOM changes inside this, and the browser animates the transition.
Imagine taking a “before photo”, making changes to the room (DOM), then taking an “after photo” — and animating between the two.
[Docs - document.startViewTransition]
@view-transition { navigation: auto;}
- A new CSS at-rule.
- Automatically applies view transitions to page changes.
- You can also add view transition names to elements to animate them individually.
- Use whenever I have lists with filters etc.
view-transition-name
- A new HTML attribute.
- Add this to any element to animate it during a view transition.
There’s a lot more to this API, including new CSS Pseudo-selectors, but this will do for now. Thanks again to the elders of the web for yet another native feature.